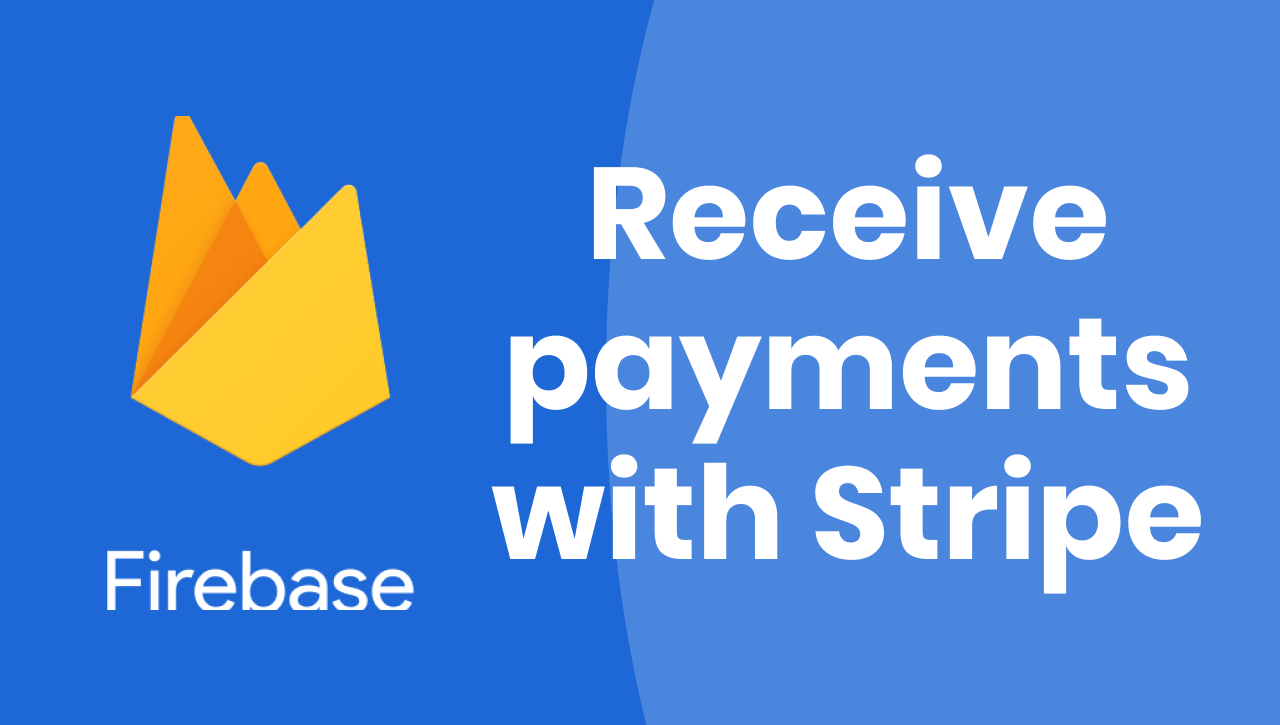
GitHub repository: https://github.com/EnterFlash/firebase-stripe
Stripe Checkout creates a secure payment page to collect payments quickly. One of the best things is that you don't have to deal with a lot of code. Stripe handles the updates in their SDK, adds multiple payment methods, takes care of things like 3D secure, and makes the web look responsive and customizable from your account's dashboard.
What is a Firebase Function?
A Firebase Function is a piece of server-side code that runs every time there's a specific trigger.
Firebase includes a client SDK to get information from databases like Firestore. However, for more complex server-side tasks, we can use Functions using Node.js, Python, or Go.
For example, you can deploy a Function that creates a Stripe Customer every time a user signs up in Firebase Authentication.
Setting up tools
Install Node and NPM as your package manager. There are different ways of doing this depending on what operating system you are using. You can use Homebrew if you are on Mac / Linux, or you can access the Node.js download page and go from there: https://nodejs.org/en/
Once we have installed NPM, we are going to use it to install the Firebase CLI tools needed to initialize our project with Firebase services (in this case, we are going to use it for Functions).
To install Firebase Tools run this command in your terminal:
$ npm install -g firebase-tools
As my text editor, I'm going to be using Visual Studio Code: https://code.visualstudio.com/
Setting up files
We are not going to use any frameworks. Except for maybe Bootstrap, everything else is going to be pure HTML, CSS, and Javascript, so go ahead and create a folder for your project, and add these files:
img/ (folder)
camera.jpg (Example picture of a camera)
index.html
scripts.js
styles.css
In our index.html file, we are going to create a simple HTML 5 structure (tip: in VS code, type "html:5" and hit TAB.)
Then, include the scripts needed for Firebase, Firebase Functions, Stripe, and Bootstrap. Also, add a simple product card with a Checkout button like this:
<!DOCTYPE html><html lang="en"><head> <title>Stripe Checkout Sample</title> <link rel="stylesheet" href="/styles.css"></head><body> <div class="card"> <img src="/img/camera.jpg" alt="Camera"> <p>New Camera</p> <p class="price">$100.00</p> <button id="checkout-button">Checkout</button> </div> <script src="https://www.gstatic.com/firebasejs/8.2.9/firebase-app.js"></script> <script src="https://www.gstatic.com/firebasejs/8.2.9/firebase-functions.js"></script> <script src="https://js.stripe.com/v3/"></script> <script src="/scripts.js"></script></body></html>
For the styles.css file, I'm using this styles:
body { font-family: sans-serif;}.card { margin: 100px auto; width: 30%; padding: 10px; text-align: center; box-shadow: 0 5px 15px #00000029; border-radius: 15px;}.card img { width: 100%; border-radius: 15px; margin-bottom: 10px;}.card p { font-weight: bold; margin: 0px 0px 5px 0px; font-size: 16pt;}.card p.price { font-weight: normal; color: #a5a5a5; margin: 0px 0px 20px 0px; font-size: 12pt;}.card button { width: 100%; height: 30px; border-radius: 15px; color: white; background-color: #3e7dff; border: none; padding: 5px 0px 5px 0px; font-weight: bold; text-transform: uppercase; letter-spacing: 1px; font-size: 8pt; cursor: pointer;}
You should now be able to open your index.html file in your browser and see a product card there.